MeasureSpec
MeasureSpec 代表的是一个 32 位的 int 值,高2位代表 SpecMode,低 30 位代表 SpecSize,SpecMode 指的测量模式,SpecSize 是指在某种测量模式下规格大小,MeasureSpec 一般在 View 的测量过程中,会比较常见。
MeasureSpec 是 View 的一个内部类,以上解释,通过以下代码可以印证上面的那段话。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
public static class MeasureSpec {
private static final int MODE_SHIFT = 30;
private static final int MODE_MASK = 0x3 << MODE_SHIFT;
public static final int UNSPECIFIED = 0 << MODE_SHIFT;
public static final int EXACTLY = 1 << MODE_SHIFT;
public static final int AT_MOST = 2 << MODE_SHIFT;
public static int makeMeasureSpec(@IntRange(from = 0, to = (1 << MeasureSpec.MODE_SHIFT) - 1) int size,@MeasureSpecMode int mode) {
if (sUseBrokenMakeMeasureSpec) {
return size + mode; // MeasureSpec 是 size + mode 组成的一个 int 值
} else {
return (size & ~MODE_MASK) | (mode & MODE_MASK);
}
}
// 转换获取测量模式
public static int getMode(int measureSpec) {
//noinspection ResourceType
return (measureSpec & MODE_MASK);
}
// 转换获取测量大小
public static int getSize(int measureSpec) {
return (measureSpec & ~MODE_MASK);
}
}
|
测量模式有 3 类,每一种都代表了不同的含义
UNSPECIFIED 父容器不对 View 有任何的限制,要多大给多大,这种情况一般是用于系统的内部,表示一种测量的状态
EXACTLY 精确模式,一般对应于 LayoutParams 中的 match_parent 和具体的数值这两种模式
AT_MOST 最大模式,一般对应于 LayoutParams 中的 wrap_content
MeasureSpec 和 LayoutParams 的对应关系
一般我们会说,如果我们设置布局的 LayoutParams 为 match_parent , 那么代表的就是的测量模式 SpecMode 就是 EXACTLY ,其实在某些情况下不是。当前 View 的测量规格是由当前 View 的 LayoutParams 和父 View 的测量规格所决定的。
但是这里还有一种情况,那就是普通 View 和顶级的 DecorView 的区别
DecorView 其测量规格是由窗口的尺寸大小和自身的 LayoutParams 来决定的
普通 View 其测量规格是由父布局的测量规格和自身的 LayoutParams 来决定的
DecorView 的测量规格
其测量规格是由窗口的尺寸大小和自身的 LayoutParams 来决定的, 在 ViewRootImpl 中可以看到如下代码
1
2
3
|
childWidthMeasureSpec = getRootMeasureSpec(desiredWindowWidht, lp.width);
childHeightMeasureSpec = getRootMeasureSpec(desiredWindowHeight, lp.height);
performMeasure(childWidthMeasureSpec, childHeightMeasureSpec);
|
其中 desiredWindowWidht 和 desiredWindowHeight 代表的就是屏幕的宽高尺寸
普通 View 的测量规格
普通 View 也就是我们自己设置的布局, View 的测量过程由 ViewGroup 中传递而来,可以看一下 ViewGroup 中的 measureChildWithMargins 源码
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
protected void measureChildWithMargins(View child,
int parentWidthMeasureSpec, int widthUsed,
int parentHeightMeasureSpec, int heightUsed) {
final MarginLayoutParams lp = (MarginLayoutParams) child.getLayoutParams();
final int childWidthMeasureSpec = getChildMeasureSpec(parentWidthMeasureSpec,
mPaddingLeft + mPaddingRight + lp.leftMargin + lp.rightMargin
+ widthUsed, lp.width);
final int childHeightMeasureSpec = getChildMeasureSpec(parentHeightMeasureSpec,
mPaddingTop + mPaddingBottom + lp.topMargin + lp.bottomMargin
+ heightUsed, lp.height);
child.measure(childWidthMeasureSpec, childHeightMeasureSpec);
}
|
可以看到,这里传递过来了父 View 的测量规格 parentWidthMeasureSpec 和 parentHeightMeasureSpec, 然后获取了自身的 LayoutParams ,然后通过了 getChildMeasureSpec 方法获取了子 View 的测量规格,可以看看 getChildMeasureSpec 方法都干了什么 ?
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
|
public static int getChildMeasureSpec(int spec, int padding, int childDimension) {
// 子 View 设置的宽高 childDimension
// 父 View 的测量模式
int specMode = MeasureSpec.getMode(spec);
// 父 View 的测量大小
int specSize = MeasureSpec.getSize(spec);
// 父 View 布局所剩余的最大空间
int size = Math.max(0, specSize - padding);
int resultSize = 0;
int resultMode = 0;
switch (specMode) {// 父 View 的测量模式
// Parent has imposed an exact size on us
case MeasureSpec.EXACTLY:
if (childDimension >= 0) {
// 如果父 View 的测量规格是精确模式,子 view 设置的是绝对数值,那么子 View 也是精确模式,子 View 大小为布局宽高
resultSize = childDimension;
resultMode = MeasureSpec.EXACTLY;
} else if (childDimension == LayoutParams.MATCH_PARENT) {
// Child wants to be our size. So be it.
// 如果父 View 的测量规格是精确模式,子 View 设置的LayoutParams 是 MATCH_PARENT,那么子 View 的测量规格是精确模式,大小为父布局所剩余的大小
resultSize = size;
resultMode = MeasureSpec.EXACTLY;
} else if (childDimension == LayoutParams.WRAP_CONTENT) {
// Child wants to determine its own size. It can't be
// 如果父 View 的测量规格是精确模式,子 View 设置的LayoutParams 是 WRAP_CONTENT,那么子 View 的测量模式是 AT_MOST,大小为父布局剩余的大小
resultSize = size;
resultMode = MeasureSpec.AT_MOST;
}
break;
// Parent has imposed a maximum size on us
case MeasureSpec.AT_MOST:
if (childDimension >= 0) {
// Child wants a specific size... so be it
// 如果父 View 的测量规格是最大模式,子 view 设置的是绝对数值,那么子 View 是精确模式,大小为子 View 设置的大小
resultSize = childDimension;
resultMode = MeasureSpec.EXACTLY;
} else if (childDimension == LayoutParams.MATCH_PARENT) {
// Child wants to be our size, but our size is not fixed.
// 如果父 View 的测量规格是最大模式,子 View 设置的LayoutParams 是 MATCH_PARENT,那么子 View 是最大模式,大小为父 View 剩余的大小
resultSize = size;
resultMode = MeasureSpec.AT_MOST;
} else if (childDimension == LayoutParams.WRAP_CONTENT) {
// Child wants to determine its own size. It can't be
// 如果父 View 的测量规格是最大模式,子 View 设置的LayoutParams 是 WRAP_CONTENT,那么子 View 是最大模式,大小为父 View 剩余的大小
resultSize = size;
resultMode = MeasureSpec.AT_MOST;
}
break;
// Parent asked to see how big we want to be
case MeasureSpec.UNSPECIFIED: // 一般情况下用于系统内部
if (childDimension >= 0) {
// Child wants a specific size... let him have it
resultSize = childDimension;
resultMode = MeasureSpec.EXACTLY;
} else if (childDimension == LayoutParams.MATCH_PARENT) {
// Child wants to be our size... find out how big it should
// be
resultSize = View.sUseZeroUnspecifiedMeasureSpec ? 0 : size;
resultMode = MeasureSpec.UNSPECIFIED;
} else if (childDimension == LayoutParams.WRAP_CONTENT) {
// Child wants to determine its own size.... find out how
// big it should be
resultSize = View.sUseZeroUnspecifiedMeasureSpec ? 0 : size;
resultMode = MeasureSpec.UNSPECIFIED;
}
break;
}
//noinspection ResourceType
return MeasureSpec.makeMeasureSpec(resultSize, resultMode);
}
|
在上面的代码中,已经写了很多的注释,详细解析看源码,相信都可以看的明白,这里再折回抛个问题,假如你在布局中设置的 LayoutParams 为 MATCH_PARENT 它就一定是 EXACTLY 精确模式吗? 仔细阅读上面的源码就会有答案 ?
这里再贴个开发中,我们平常遇到的情况,结合上面源码部分,就可以知道答案。
这里为什么设置的是 wrap_content ,布局的内容确是填充了整个屏幕 ?
因为父 View 的测量规格是精确模式,子 View 设置的 LayoutParams 是 WRAP_CONTENT,那么子 View 的测量模式是 AT_MOST,大小为父布局剩余的大小,这里的父 View 指的是 DocerView
或者是下面这种情况,也是一样的,这也就解释了,为什么设置的是 wrap_content 为什么填满了整个窗口。
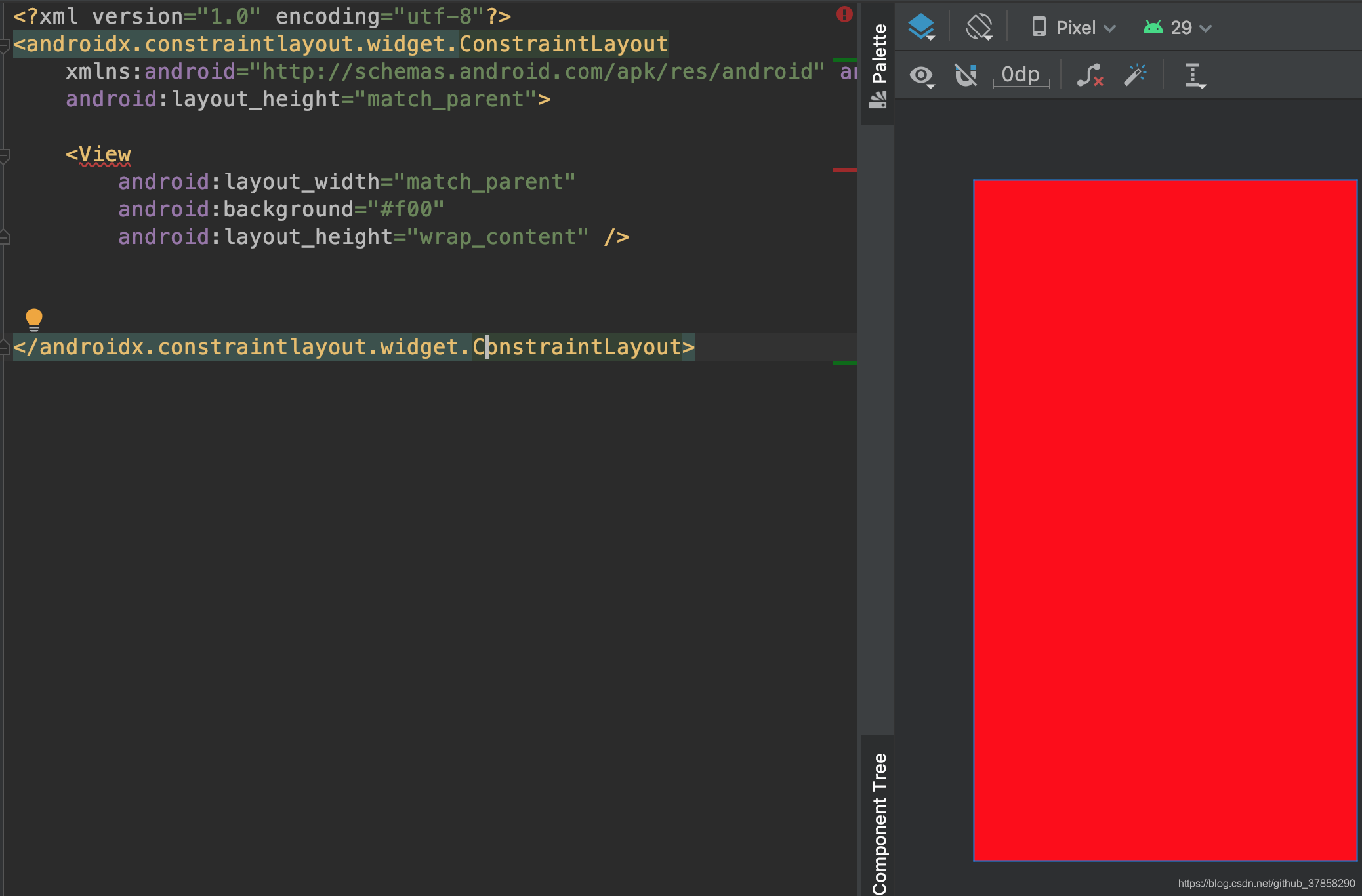